- Pure POJO action beans with zero framework dependencies
- Annotation-based dependency injection (typed request parameters, session attributes, Spring beans, and many others)
- Converters and validators type-parameterized using Java 5 generics
- Mechanisms for facilitating use of redirect after post pattern
- Support for rendering using Spring MVC Views and View Resolvers
- Pre- and post- action interceptors, with access to dependency resolved action beans as well as full runtime context
- Works on the unchanged Struts 1.2.x and 1.3.x code bases.
- Actions, form validation and data conversion easily testable with plain unit tests, with no additional test libraries required.
Monday, October 30, 2006
Strecks 1.0 released
Strecks a set of Java 5-specific extensions Struts framework, was released from beta on friday. Strecks, is annotation based and adds a number of modern features to Struts-based applications, including POJO actions, dependency injection, declarative validation and data binding, interceptors, pluggable views, as well as seamless Spring integration. It is also highly extensible and amenable to test driven development. The following is a brief list of features available in Strecks 1.0:
Friday, October 27, 2006
Struts 2: Info and Resources
Struts has been the most popular web application framework for the past few years. With this popularity have come enhancements and changes due not only to the changing requirements, but also to provide features available in newer frameworks. The new version Struts 2.0 is a combination of the Sturts action framework and Webwork. According to the Struts 2.0.1 release announcement, some key changes are:
- Improved Design - All Struts 2 classes are based on interfaces. Core interfaces are HTTP independent.
- Intelligent Defaults - Most configuration elements have a default value that we can set and forget.
- Enhanced Results - Unlike ActionForwards, Struts 2 Results can actually help prepare the response.
- Enhanced Tags - Struts 2 tags don't just output data, but provide stylesheet-driven markup, so that we can create consistent pages with less code.
- First-class AJAX support - The AJAX theme gives interactive applications a significant boost.
- Stateful Checkboxes - Struts 2 checkboxes do not require special handling for false values.
- QuickStart - Many changes can be made on the fly without restarting a web container.
- Easy-to-test Actions - Struts 2 Actions are HTTP independent and can be tested without resorting to mock objects.
- Easy-to-customize controller - Struts 1 lets us customize the request processor per module, Struts 2 lets us customize the request handling per action, if desired.
- Easy-to-tweak tags - Struts 2 tag markup can be altered by changing an underlying stylesheet. Individual tag markup can be changed by editing a FreeMarker template. No need to grok the taglib API! Both JSP and FreeMarker tags are fully supported.
- Easy cancel handling - The Struts 2 Cancel button can go directly to a different action.
- Easy Spring integration - Struts 2 Actions are Spring-aware. Just add Spring beans!
- Easy plugins - Struts 2 extensions can be added by dropping in a JAR. No manual configuration required!
- POJO forms - No more ActionForms! We can use any JavaBean we like or put properties directly on our Action classes. No need to use all String properties!
- POJO Actions - Any class can be used as an Action class. We don't even have to implement an interface!
Wednesday, October 25, 2006
Firefox 2.0 released
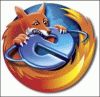
Last week, Microsoft released the new Internet Explorer 7.0. Today it is the release of Mozilla Firefox 2.0. Which one to choose? Well, for me it's Firefox for sure. For one thing, I still run windows 2000 at work.(IE 7 does not support windows 2000). More importantly, IE 7 introduces many security enhancements in the new product, which I will be among the last to try, given Microsoft's record on security. Coming to Firefox 2, there are no big changes that you were not available as addons in the earlier version. I still hate the fact that it uses up so much memory. Agreed that I have a few addons (greasemonkey, NoScript, Firebug, and web developer toolbar), but still the startup memory consumption in firefox is 25MB without addons and about 35MB with addons. As you can see in the picture below, my regular usage goes up to 246MB regularly. I never saw anything like that with IE 6, even with over over 10 windows open at a time (about the same number of tabs were open in firefox when I took the snapshot.
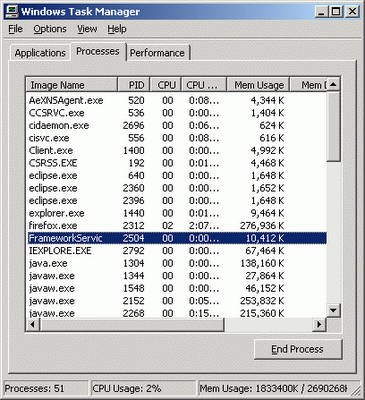
Returning data from anonymous PL/SQL block
The example here demonstrates the use of an anonymous PL/SQL block to return data to a calling Java program. It also shows how to use nested tables in PL/SQL, and the use of auto-generated column values. This requires Java 5 and Oracle 10g Release 2. In order to use the example, on the database
This code was tested on Java 5.0 with Oracle 10g Release 2.
References:
- Create a trigger on the database table that inserts a value into the new row, or use the following piece of code before the insert statement and insert the seqval variable in the id column.
select req_seq.nextval into seqval from dual;
Here req_seq is a sequence holding the req_id sequence. - Create two new types as shown below
create or replace TYPE RQ_ROW AS TABLE OF VARCHAR(500);
create or replace TYPE RQ_LIST AS TABLE OF RQ_ROW;
create or replace TYPE RESULTS_LIST AS TABLE OF RESULT_LIST;
create or replace TYPE RESULT_LIST AS VARRAY OF NUMBER;
RQ_ROW represents a row in the table. RQ_LIST represents a set of rows. RESULT_LIST is a two element list where the first element represents the index and the second element is the autogenerated column value. This was used since nested tables do not guarantee the order of elements. RESULTS_LIST is used to return the autogenerated keys back to Java.
rqList is the input parameter here. Note the ? := rqResults; This represents the output parameter. The following code snippet shows the implementation of this in a Java program.
DECLARE
rqResults RESULTS_LIST;
rqRow RQ_ROW;
rqList RQ_LIST;
p Number;
q Number;
BEGIN
rqList := ?;
rqresults := results_list();
q := rqList.COUNT;
FOR k IN 1..q LOOP
INSERT INTO rquisitions(RQ_CD, RQ_STATUS)
VALUES (rqList(k)(1), rqList(k)(2)) RETURNING rq_id INTO p;
rqResults.EXTEND;
rqResults(k) := RESULT_LIST(k,p);
END LOOP;
? := rqResults;
END;
Note the use of a callable statement and that the out parameter is an array. It is actually an array of arrays. The java.sql.Array class provides a getResultSet() method that can be used to browse the array elements.
private String sql = "DECLARE " +
"rqResults RESULTS_LIST; " +
"rqRow RQ_ROW; " +
"rqList RQ_LIST; " +
"p Number; " +
"q Number;" +
"BEGIN " +
"rqList := ?; " +
"rqresults := results_list(); " +
"q := rqList.COUNT; " +
"FOR k IN 1..q LOOP " +
"INSERT INTO rquisitions(RQ_CD, RQ_STATUS) VALUES " +
"(rqList(k)(1), rqList(k)(2)) RETURNING rq_id INTO p; " +
"rqResults.EXTEND; " +
"rqResults(k) := RESULT_LIST(k,p);" +
"END LOOP; " +
"? := rqResults;" +
"END;";
public void load() {
Connection connection = getConnection();
String RQ_COLS[] = { "req_id" };
try {
String data[][] = {{"ab", "cd"}, {"ef", "gh"}, {"ij", "kl"}};
ArrayDescriptor descriptor =
ArrayDescriptor.createDescriptor("SCOTT.RQ_LIST", connection);
Array array = new ARRAY(descriptor, connection, data);
CallableStatement cstmt = null;
cstmt = connection.prepareCall(sql);
cstmt.setArray(1, array);
cstmt.registerOutParameter(2, Types.ARRAY, "SCOTT.RESULTS_LIST");
cstmt.executeUpdate();
// Print the result set.
Array arr = cstmt.getArray(2);
ResultSet rSet = arr.getResultSet();
long[] values = new long [6];
while (rSet.next()) {
Array arr1 = (Array)rSet.getArray(2);
ResultSet rrSet = arr1.getResultSet();
while(rrSet.next()) {
int index = rrSet.getInt(2);
if(rrSet.next()) {
long value = rrSet.getLong(2);
values[index] = value;
}
}
}
for(int i = 1; i < values.length; i ++) {
System.out.println(i + ", " + values[i]);
}
cstmt.close();
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
This code was tested on Java 5.0 with Oracle 10g Release 2.
References:
Tuesday, October 24, 2006
Java 6: Native Platform Security
The Java Platform, Standard Edition (Java SE) provides application developers with a large set of security APIs, tools, and implementations of commonly used security algorithms, mechanisms, and protocols. A new article article titled "Leveraging Security in the Native Platform Using Java SE 6 Technology" discusses important enhancements on the native security integration using JDK 6, to enable the Java developer use the enhancements to native platforms such as, cryptographic accelerators, secure key management etc. The enhancements to provided in Java 6 include:
References:
- Access Microsoft CryptoAPI and Its Cryptographic Services: On the Microsoft (MS) Windows operating system, the MS CryptoAPI (CAPI) defines a standard interface for performing cryptographic operations as well as accessing the user keys and certificates that Windows manages. The SunMSCAPI provider is layered on top of CAPI and helps Java platform applications access CAPI cryptographic services to Access private keys and certificates stored in CAPI and Use CAPI's cryptographic algorithm implementations
- Access PKCS#11 Cryptographic Services: PKCS#11, the Cryptographic Token Interface Standard, defines native programming interfaces to cryptographic tokens such as hardware cryptographic accelerators and smart cards. This means that Java platform applications (from JDK 5) can use existing security and cryptography APIs in the Java platform to access
- Cryptographic smart cards for added security
- Hardware cryptographic accelerators for better performance
- Software implementations for more algorithms or for meeting certification requirements
- Access Native GSS-API: The Generic Security Services API (GSS-API) defines a generic security API atop a variety of underlying cryptographic mechanisms including Kerberos version 5. With GSS-API, applications can authenticate a principal, delegate its rights to a peer, and apply security services such as confidentiality and integrity on a per-message basis.
- Import and Export PKCS#12 Keystores: PKCS#12, the Personal Information Exchange Syntax Standard, defines a portable format for storing or transporting personal identity information, including private keys, certificates, and so on. This enables users to share their personal identity information among applications that support this standard. In particular, user credentials that browsers generate can be exported in PKCS#12 format and accessed and used by Java platform applications.
References:
- Leveraging Security in the Native Platform Using Java SE 6 Technology
- Java Security Overview
- Java Cryptography Architecture API Specification and Reference Guide
- Java Secure Socket Extension (JSSE) Reference Guide
- Java PKCS#11 Reference Guide
- Default Policy Implementation and Policy File Syntax
- Java GSS API (RFC 2853)
- Introduction to JAAS and Java GSS-API Tutorials
- Single Sign-on Using Kerberos in Java
- Java SASL API Programming and Deployment Guide
Thursday, October 19, 2006
AJAX in Java with DWR
Direct Web Remoting (DWR) is an engine that exposes methods of server-side Java objects to JavaScript code. With DWR, your client-side code need to use the XMLHttpRequest object to make asynchronous calls. You don't even need to write servlet code to mediate Ajax requests into calls on your Java domain objects (the way you do when using prototype.js). Follow these steps to implement Ajax in your application:
References:
- Implement a Java class that will act as the remote interface. It can be any Java class with any methods.
- Configure DWR in the WEB-INF/dwr.xml file defining the methods to be exposed as shown below.
<dwr>
<allow>
<create creator="new" javascript="JavascriptName">
<param name="class"
value="JavaClassName"/>
<include method="method1"/>
<include method="method2"/>
</create>
<convert converter="bean"
match="beanType">
<param name="include"
value="attr1,attr2,..."/>
</convert>
</allow>
</dwr> - Define the DWR Servlet in your web.xml file:
<servlet>
<servlet-name>dwr-invoker</servlet-name>
<servlet-class>uk.ltd.getahead.dwr.DWRServlet</servlet-class>
<init-param>
<param-name>debug</param-name>
<param-value>true</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>dwr-invoker</servlet-name>
<url-pattern>/dwr/*</url-pattern>
</servlet-mapping> - Invoke the methods from client-side Javascript using the notation:
JavaScriptName.methodName(methodParams ..., callBack)
Where the callBack method handles the data returned from the server. You will have to include engine.js and util.js available from the DWR site.
References:
Monday, October 16, 2006
Hibernate 3.2
The Hibernate developer team released Hibernate 3.2.0 GA, this release is certified compliant with the Java Persistence API. In addition to JPA compliance, hibernate adds new query capabilities, declarative data filters, Hibernate Annotations, Hibernate EntityManager and optimistic locking in a cluster with JBoss Cache. The Hibernate Java Persistence provider has been certified with the Sun TCKThe Hibernate 3.2 release includes:
- Natural programming model - Hibernate supports natural OO idiom; inheritance, polymorphism, composition and the Java collections framework
- Support for fine-grained object models - a rich variety of mappings for collections and dependent objects
- No build-time bytecode enhancement - there's no extra code generation or bytecode processing steps in your build procedure
- Extreme scalability - Hibernate is extremely performant, has a dual-layer cache architecture, and may be used in a cluster
- The query options - Hibernate addresses both sides of the problem; not only how to get objects into the database, but also how to get them out again
- Support for "conversations" - Hibernate supports both long-lived persistence contexts, detach/reattach of objects, and takes care of optimistic locking automatically
- Free/open source - Hibernate is licensed under the LGPL (Lesser GNU Public License)
- EJB 3.0 - Hibernate implements the Java Persistence management API and object/relational mapping options, two members of the Hibernate team are active in the expert group
- Hibernate Annotations offers several packages of JDK 5.0 code annotations that developers can use to map classes, as a replacement or in addition to XML metadata.
Tibco GI now opensource
TIBCO General Interface is a AJAX rich internet application (RIA) toolkit that lets organizations capitalize on the lower costs of Web applications while delivering the rich graphical look and feel of desktop-installed software and components. General Interface helps you in creating sophisticated web-based applications that run in a standard web browser without plug-ins, Active-X controls, Java applets or client-side software installation. Now, Tibco released Tibco General Interface (GI) 3.2 Beta, which is opensourced under the BSD license. Tibco GI, version 3.2 introduces several major features:
- New BSD license
- Firefox 1.5 support
- powerful new components
- New Matrix control combines Tree, List and Edit Grid capabilities and adds large data set scrolling and pagination tuners
- Chart package implemented in SVG to enable execution in Firefox without a plug-in
- Load-time optimizations with smaller initial footprint
- API and visual tooling enhancements throughout
- Lots more as described in the release notes
Thursday, October 12, 2006
Tuesday, October 03, 2006
Firefox Javascript Vulnerability?
Apparently, the vulnerability reported in the handling of Javascript in firefox is a hoax. See
Subscribe to:
Posts (Atom)
Popular Posts
-
This post will describe how to create and deploy a Java Web Application war to Heroku using Heroku CLI. You will need a basic understanding ...
-
In this post we will see how to do an offline install Jenkins and required plugins on a Red Hat Enterprise Linux Server release 7.3. This is...
-
Update: A new post for validation in struts with annotation is available at: Struts 2 Validation: Annotations . Struts 2.0 relies on a val...
-
The previous post described the Strategy pattern in brief. I listed out where and why the strategy pattern may be used. This post describes...
-
In a previous post, I described how to use Quartz scheduler for scheduling . In this post, I describe the configuration changes required for...
-
Java 8 introduced default and static methods, the previous post gives a few examples of Java 8 default and static methods . Java 9 builds on...
-
Tivoli Access Manager Configuration Steps for using AznAPI: 1.) Configure AMRTE 2.) Configure AMJRTE (This will install the necessary librar...
-
Google has opensourced it's Google Web Toolkit . The project is now fully opensource under the Apache 2.0 license . The new 1.3 RC has...
-
A class that switches between a set of states, and behaves differently based on the current state it is in, is a good candidate for the stat...
-
Previously, I wrote a post describing the use of Apache Axis to create and consume Web Services from Java . In this post, I will describe ho...