This JavaOne 2006 presentation looks at the benefits and issues of migrating to Java 1.5.
The presentation also looks at how to take advantage of the superior VM performance, security enhancements, XML support, JMX and JDBC enhancements and deployment improvements.
Wednesday, September 27, 2006
Monday, September 18, 2006
Oracle JDBC: Automatic key generation and retrieval
Oracle provides the sequence utility to automatically generate unique primary keys. In your Oracle database, you must create a sequence table that will create the primary keys, as shown in the following example:
Note:
Sample Code
The following code illustrates retrieval of auto-generated keys:
create sequence myOracleSequenceThis creates a sequences of primary key values, starting with 1, followed by 2, 3, and so forth. JDBC 3.0 introduces the retrieval of auto-generated keys feature that enables you to retrieve such generated values. In JDBC 3.0, the following interfaces are enhanced to support the retrieval of auto-generated keys feature:
start with 1
nomaxvalue;
- java.sql.DatabaseMetaData
public boolean supportsGetGeneratedKeys();
The method indicates whether retrieval of auto-generated keys is supported or not by the JDBC driver and the underlying data source. - java.sql.Statement
public boolean execute(String sql, int autoGeneratedKeys) throws SQLException;
These methods take a String object that contains a SQL statement. They also take either the flag, Statement.RETURN_GENERATED_KEYS, indicating whether any generated columns are to be returned, or an array of column names or indexes specifying the columns that should be returned. The getGeneratedKeys() method enables you to retrieve the auto-generated key fields. The auto-generated keys are returned as a ResultSet object.
public boolean execute(String sql, int[] columnIndexes) throws SQLException;
public boolean execute(String sql, String[] columnNames) throws SQLException;
public boolean executeUpdate(String sql, int autoGeneratedKeys) throws SQLException;
public boolean executeUpdate(String sql, int[] columnIndexes) throws SQLException;
public boolean executeUpdate(String sql, String[] columnNames) throws SQLException;
public ResultSet getGeneratedKeys() throws SQLException;
When the Statement.RETURN_GENERATED_KEYS integer flag is used, Oracle JDBC drivers cannot identify these columns, since the column indices/names have not been specified. Therefore, when the integer flag is used to indicate that auto-generated keys are to be returned, the ROWID pseudo column is returned as key. The ROWID can be then fetched from the ResultSet object and can be used to retrieved other columns. - java.sql.Connection
public PreparedStatement prepareStatement(String sql, int autoGeneratedKeys) throws SQLException;
These methods enable you to create a PreparedStatement object that is capable of returning auto-generated keys.
public PreparedStatement prepareStatement(String sql, int[] columnIndexes) throws SQLException;
public PreparedStatement prepareStatement(String sql, String[] columnNames) throws SQLException;
Note:
- This feature is supported only when INSERT statements are processed. Other data manipulation language (DML) statements are processed, but without retrieving auto-generated keys.
- The Oracle server-side internal driver does not support the retrieval of auto-generated keys feature.
- This feature is only available since Oracle Database 10g Release 2.
Sample Code
The following code illustrates retrieval of auto-generated keys:
/** SQL statements for creating an ORDERS table and a sequence for generating theReferences:
* ORDER_ID.
*
* CREATE TABLE ORDERS (ORDER_ID NUMBER, CUSTOMER_ID NUMBER, ISBN NUMBER,
* DESCRIPTION NCHAR(5))
*
* CREATE SEQUENCE SEQ01 INCREMENT BY 1 START WITH 1000
*/
...
String cols[] = {"ORDER_ID", "DESCRIPTION"};
// Create a PreparedStatement for inserting a row in to the ORDERS table.
OraclePreparedStatement pstmt = (OraclePreparedStatement) conn.prepareStatement
("INSERT INTO ORDERS (ORDER_ID, CUSTOMER_ID, ISBN,
DESCRIPTION) VALUES (SEQ01.NEXTVAL, 101, 966431502,
?)", cols);
char c[] = {'a', '\u5185', 'b'};
String s = new String(c);
pstmt.setFormOfUse(1, OraclePreparedStatement.FORM_NCHAR);
pstmt.setString(1, s);
pstmt.executeUpdate();
ResultSet rset = pstmt.getGeneratedKeys();
Wednesday, September 13, 2006
java.text.Collator for String Comparison
The String class doesn't have the ability to compare text from a natural language perspective. Its equals and compareTo methods compare the individual char values in the string. If the char value at index n in name1 is the same as the char value at index n in name2 for all n in both strings, the equals method returns true. The java.text.Collator class provides natural language comparisons. Natural language comparisons depend upon locale-specific rules that determine the equality and ordering of characters in a particular writing system.A Collator object understands that people expect "cat" to come before "Hat" in a dictionary. Using a collator comparison, the following code prints cat < Hat.
Collator collator = Collator.getInstance(new Locale("en", "US"));For a detailed description and extra information refer to Strings - Core Java Technologies Technical Tips.
int comparison = collator.compare("cat", "Hat");
if (comparison < 0) {
System.out.printf("%s < %s\n", "cat", "Hat");
} else {
System.out.printf("%s < %s\n", "Hat", "cat" );
}
Tuesday, September 12, 2006
Notes: Declarative Authentication in JEE
A Java EE web-application can be configured to restrict access to resources via the deployment descriptor (web.xml). Authentication does not need any programming, as it is handled by the container (provided it is properly configured). Here are two ways to implement declarative authentication in Java EE web applications:
Basic Authentication
The web.xml snippet below uses BASIC authentication:
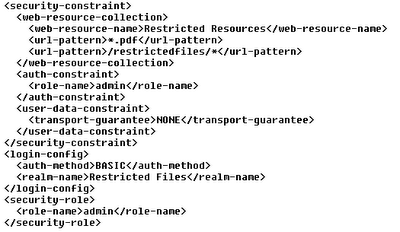
Form Authentication
In the snippet below, the section has been changed to use FORM authentication. This will require an additional login form to be created with j_security_check as it's action.

The following is the sample form:

Other possible values for are DIGEST and CLIENT-CERT.The value of essentially defines whether SSL is required or not. If the value is INTEGRAL or CONFIDENTIAL, then you can assume that an https request is required to access the resource.
Basic Authentication
The web.xml snippet below uses BASIC authentication:
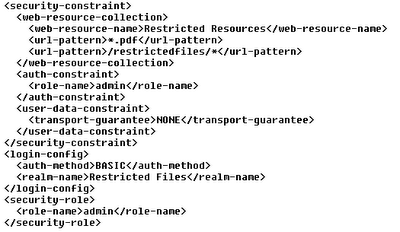
Form Authentication
In the snippet below, the

The following is the sample form:

Other possible values for
Friday, September 01, 2006
Eclipse SQL Explorer
Eclipse SQL Explorer 3, an Eclipse plugin that allows you to query and browse any JDBC compliant database, has been released. The SQL Explorer adds a new perspective and a few new views to eclipse. The following is a short list:
- The SQL editor provides syntax highlighting and content assist.
- Using the Database Structure view, you can explore multiple databases simultaneously. When a node is selected, the corresponding detail is shown in the database detail view.
- Provides database-specific features for DB2, Oracle, and MySQL)
Subscribe to:
Posts (Atom)
Popular Posts
-
JUnit 4 introduces a completely different API to the older versions. JUnit 4 uses Java 5 annotations to describe tests instead of using in...
-
In a previous post, I described how to use Quartz scheduler for scheduling . In this post, I describe the configuration changes required for...
-
Sun Microsystems, Inc. announced the availability of Java Platform Standard Edition 6 ( Java SE 6 ) today. In less than 2yrs of the release ...
-
In this post we will see a way to merge multiple PDF files while adding page numbers at the bottom of each page in the format Page 1 of 10 ....
-
New posts with iText 5.5.12 Following are two new posts for PDF Merge with iText 5.5.12 Merge PDF files using iText 5 Merge and Paginate PDF...
-
The struts pagination post contained an example of using Hibernate for data access too. In this post, I use the same example to implement th...
-
The previous post described how to implement a JMS messaging client using Spring JMS . This post will describe how to implement the Message ...
-
In this post we will see how to do an offline install Jenkins and required plugins on a Red Hat Enterprise Linux Server release 7.3. This is...
-
OTN's "Greatest Hits" CD is a compilation of the most popular technical articles, software downloads, podcasts, sample code, ...
-
In the past, I had a few posts on how to implement pagination using displaytag( 1 , 2 ). That solution is feasible only with small result se...